- Compatible API Versions
- 1.0.0 and higher
- Contributors
- Creadores Program & RedstoneAlmeida
JSEngineNK
Nukkit plugin, enable to load javascript! very simple create systems!!
With the Power of the Nashorn Engine!
Nukkit plugin
- Allows you to load Javascript modules! Runs ES6 version (Some things are with polyfills, especially classes, see how they are created) In addition to the fact that the other versions of JavaScript are in Partial
Credits to the Original Plugin: https://cloudburstmc.org/resources/modloader.108/
How to use?
Create javascript archive, example: mod.js
Drop archive in plugins/JSEngineNK/mod.js
Create your code!
Start your server! Instantly run
examples can be found (here) [https://github.com/Trollhunters501/JSEngineNK/tree/master/examples]
Basic JavaScript API loaded!:
Global Variables:
Create Basic Command:
Create Basic Tasks (The second condition of the function is the time to wait in ticks In Minecraft):
Run Events:
Create Config:
Register Script:
Using Packages from nukkit and Java Vanila:
View scripts by name I recommend using when finished loading all the scripts!):
Isolated or Private functions: (variables or functions will not be global Neither events nor tasks can be registered):
Import external libraries from java by Maven:
Import external libraries JS by URL:
To see all manager functions go to [https://github.com/Trollhunters501/JSEngineNK/blob/master/Manager info/ManagerFunc.md]
How to create JavaScript Classes:
Extend Java classes:
Default libraries in the plugin:
Fetch: https://raw.githubusercontent.com/Trollhunters501/Fetch-API-Nashorn/main/Creadores Program/Nashorn NK/Fetch API.js
Normalize Polyfill: https://cdn.jsdelivr.net/npm/[email protected]/lib/unorm.min.js
UnderScoreJS: https://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.13.6/underscore-min.js
Loadash: https://cdn.jsdelivr.net/npm/lodash@4/lodash.min.js
RequireAPI: https://raw.githubusercontent.com/pldrjs/jvm-npm/ad122db2bd7f55156e2dc86115845efae6d13ec5/src/main/javascript/jvm-npm.js
Base64: https://raw.githubusercontent.com/Trollhunters501/JSEngineNK/master/libs/base64.js
Adder: https://raw.githubusercontent.com/maheshrajannan/java8Nashorn2/master/src/main/java/java8Group/java8Artifact/js/adder.js
NnClassLoader: https://cdn.rawgit.com/NashornTools/NnClassLoader/master/NnClassLoader.js
ES6 from Nashorn: https://raw.githubusercontent.com/aesteve/nashorn-es6/master/src/main/resources/es6-shim.js
Class from Nashorn: https://raw.githubusercontent.com/Trollhunters501/JSEngineNK/master/libs/Clases.js
Polyfills from Nashorn Extra: https://raw.githubusercontent.com/Trollhunters501/JSEngineNK/master/libs/NewPolifills.js
If you want to make plugins for your server, do not hesitate to Contact Us!
Discord: https://discord.gg/mrmHcwxXff
WhatsApp channel: https://whatsapp.com/channel/0029Va5bITcGZNCqyYkDYZ0F
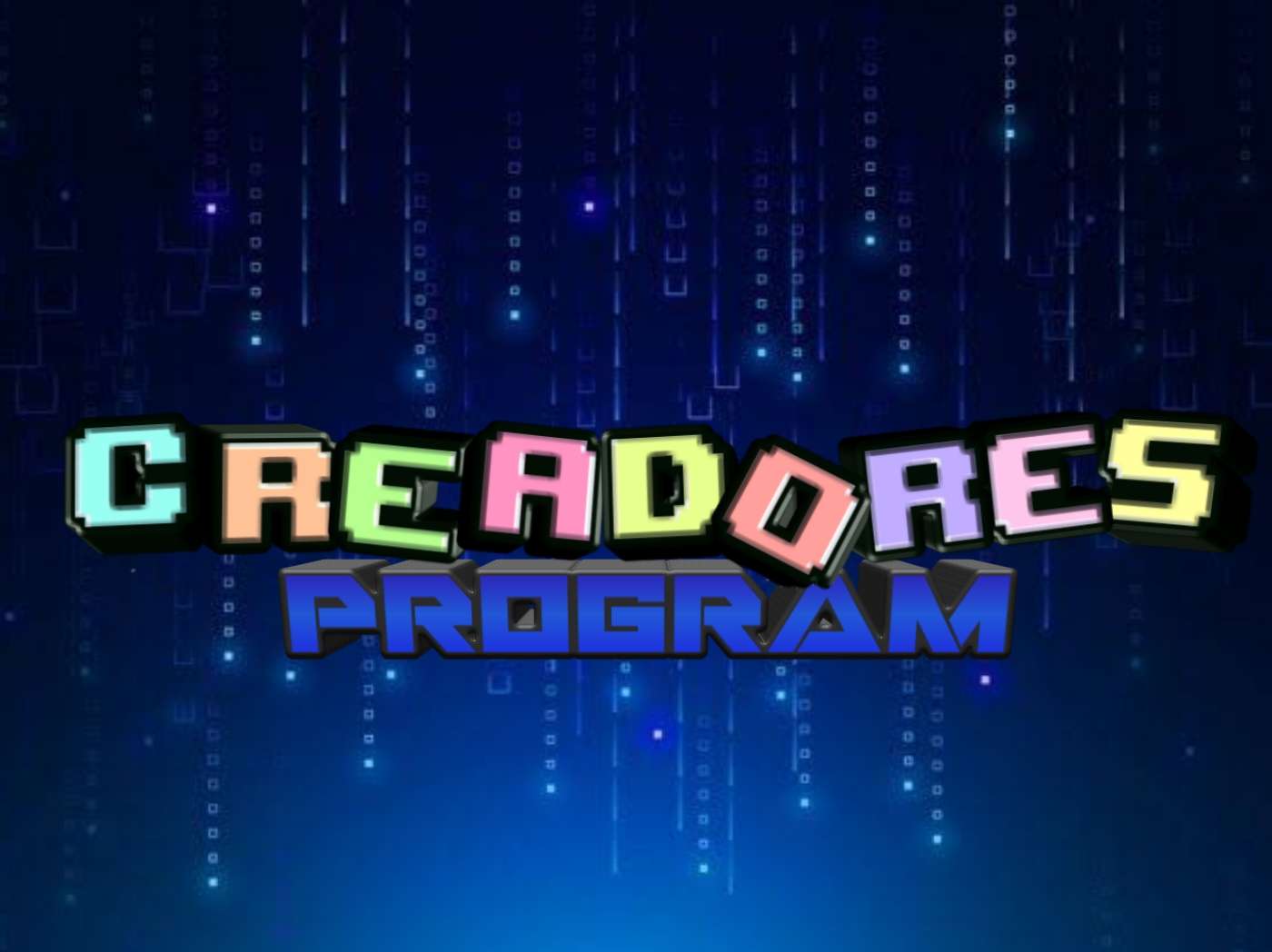
2024©
Nukkit plugin, enable to load javascript! very simple create systems!!
With the Power of the Nashorn Engine!
Nukkit plugin
- Allows you to load Javascript modules! Runs ES6 version (Some things are with polyfills, especially classes, see how they are created) In addition to the fact that the other versions of JavaScript are in Partial
Credits to the Original Plugin: https://cloudburstmc.org/resources/modloader.108/
How to use?
Create javascript archive, example: mod.js
Drop archive in plugins/JSEngineNK/mod.js
Create your code!
Start your server! Instantly run
examples can be found (here) [https://github.com/Trollhunters501/JSEngineNK/tree/master/examples]
Basic JavaScript API loaded!:
Global Variables:
JavaScript:
var server; return getServer();
var plugin; return JSEngineNK Plugin MainClass;
var global; return JSEngineNK Plugin MainClass;
var self; return JSEngineNK Plugin MainClass;
var manager; return FunctionManager Class, Using to create Commands e Loops
var script; return A class that registers scripts and events
var logger; return Console Logger Input
var console; return Console Logger Input
var window; return Console Logger Input
var players; return All Online Players
JavaScript:
manager.createCommand("name", "description", "functionUsed", "usage", ["aliase1", "aliase2"], "perm.nk");
function functionUsed(sender, args, label, manageCMD){
if(args.length < 1){ // see args exists
sender.sendMessage('You used incorrect!');
return;
}
let name = args[0];
sender.sendMessage("You writer: " + name); // send Message to sender
// sender.sendMessage(manager.format("You writer: %s", name)); //format your message
}
JavaScript:
manager.createTask("tasknormal", 20);
manager.createLoopTask("taskloop", 20);
function tasknormal(currentTick){
print('I tasknormal!');
}
function taskloop(currentTick){
print('I taskloop');
}
JavaScript:
script.addEventListener("PlayerJoinEvent", function(event){
let player = event.getPlayer();
player.sendMessage("welcome to Server!");
});
// ready, start your server and test!
JavaScript:
var config = manager.createConfig(manager.getFile("folder", "archive"), 2); // 2 = Config.YAML
config.set("key", "value");
config.save();
JavaScript:
script.registerScript({
name: "TestScript",
version: "1.0",
description: "The Test!",
website: "https://github.com/Trollhunters501/JSEngineNK/",
authors: ["Creadores Program & RedstoneAlmeida"]
});
//You can register your script so that it appears in the list of scripts with the command /scripts or also with the command /version script or /ver script
//The mandatory parameters are: author or authors, name, version and description optional: website
JavaScript:
var playertest = Java.type("cn.nukkit.Player");
//The Player file you can see the Nukkit API in their official documentation on how to use the files! (I recommend using hard to replicate variables as another script may use the same variable)
var IOExeptionTest = Java.type("java.io.IOException");
//java vanilla
var ContentType = Packages.org.apache.http.entity.ContentType;
//Packages which are not in Java Vanila or Nukkit (Necessary to install the Packages )
JavaScript:
script.getScriptByName("here the name of the script!");
JavaScript:
//These are isolated functions that means that the variables and functions inside it cannot be executed and neither can events nor tasks be registered!
(function(){
//code...
})();
JavaScript:
//import libraries from java by Maven:
// Define Maven dependencies for the script
var MAVEN_DEPENDENCIES = ['com.h2database:h2:1.4.192', 'org.apache.commons:commons-dbcp2:2.1.1'];
// Create class loader instance.
var L = new NnClassLoader({ maven: MAVEN_DEPENDENCIES });
// Look at the actual list of jars resolved by the class loader.
for each(var url in L.getUrls()) print(url);
// Import class similarly to Java.type
var BasicDataSource = L.type('org.apache.commons.dbcp2.BasicDataSource');
// Work with imported classes as usual
var ds = new BasicDataSource();
// ...
JavaScript:
//How to import a javascript file from a web page:
load("https://example.com/exam.js");
//Done!
How to create JavaScript Classes:
JavaScript:
//You must create a const variable with the Class() function and with 2 parameters The name of the variable will be the name of your class!
//The first parameter is always Object except if you want to extend another JavaScript class In that case just change Object to the name of the class (The class should have already been established)
//The second parameter is the class functions!(If you extend any class, for no reason do you give your functions the same name as the extended classes (The JavaScript engine gets confused and crashes))
const Says = Class(Object, {
say: function(){
console.info('Hello');
}
});
//Create a call to the class:
var Hey = new Says();
//Call the function:
Hey.say();
//Output: Hello
//Extend a Class:
//Extends the previous class
const Greeting = Class(Says, {
//Respecting that the function is not repeated, in this case you cannot repeat say()
hi: function(){
//Call to the class that extends:
this.say();
//Plus extra code
console.info('Console!');
}
});
//We call the class the same as before
var HiCon = new Greeting();
HiCon.hi();
//Output: Hello
//Console!
//Done!
JavaScript:
var ClassExample = Java.type("class.extend.java");
var extendC = Java.extend(ClassExample); //Also works with classes imported with the Nnclassloader library
var myclassextend = new extendC(){
onEnable: function(){
aFunctionOfTheExtendedClass("hello!");
}//Useful for using @ in JSEngineNK without coding directly in Java and leaving your class empty!
}
Fetch: https://raw.githubusercontent.com/Trollhunters501/Fetch-API-Nashorn/main/Creadores Program/Nashorn NK/Fetch API.js
Normalize Polyfill: https://cdn.jsdelivr.net/npm/[email protected]/lib/unorm.min.js
UnderScoreJS: https://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.13.6/underscore-min.js
Loadash: https://cdn.jsdelivr.net/npm/lodash@4/lodash.min.js
RequireAPI: https://raw.githubusercontent.com/pldrjs/jvm-npm/ad122db2bd7f55156e2dc86115845efae6d13ec5/src/main/javascript/jvm-npm.js
Base64: https://raw.githubusercontent.com/Trollhunters501/JSEngineNK/master/libs/base64.js
Adder: https://raw.githubusercontent.com/maheshrajannan/java8Nashorn2/master/src/main/java/java8Group/java8Artifact/js/adder.js
NnClassLoader: https://cdn.rawgit.com/NashornTools/NnClassLoader/master/NnClassLoader.js
ES6 from Nashorn: https://raw.githubusercontent.com/aesteve/nashorn-es6/master/src/main/resources/es6-shim.js
Class from Nashorn: https://raw.githubusercontent.com/Trollhunters501/JSEngineNK/master/libs/Clases.js
Polyfills from Nashorn Extra: https://raw.githubusercontent.com/Trollhunters501/JSEngineNK/master/libs/NewPolifills.js
If you want to make plugins for your server, do not hesitate to Contact Us!
Discord: https://discord.gg/mrmHcwxXff
WhatsApp channel: https://whatsapp.com/channel/0029Va5bITcGZNCqyYkDYZ0F
2024©